Users try to access your site, you know what happened? It doesn’t show up. There are some errors that you didn’t figure out before. Users get frustrated and leave your site. So, you lost some loyal users with it.
How to solve this problem? How do you get to know about the site down before your users find it?
There are two possible ways:
If you don’t mind spending a few $, you can go for a monitoring solution like StatusCake or others mentioned here. However, if you are a developer or not ready to spend monthly, you can take advantage of Geekflare API – Is Site Up?
Is Site Up? API checks whether a site is up or down from different locations.
Let me show you Python code that notifies you immediately when your site goes down via Gmail.
Let’s start with exploring the Is Site Up? API.
Is Site Up? API
Before checking the API we need to install a package called requests to work with APIs in Python. But, it’s not necessary to use the only Python. You can use your preferred language. But, make sure you set up the required things to make an API request.
So, for those who are using Python install the requests package using the command pip install requests
Complete the setup for other languages (if you choose other than Python) and continue to the next steps.
Now, go to the Geekflare API page.
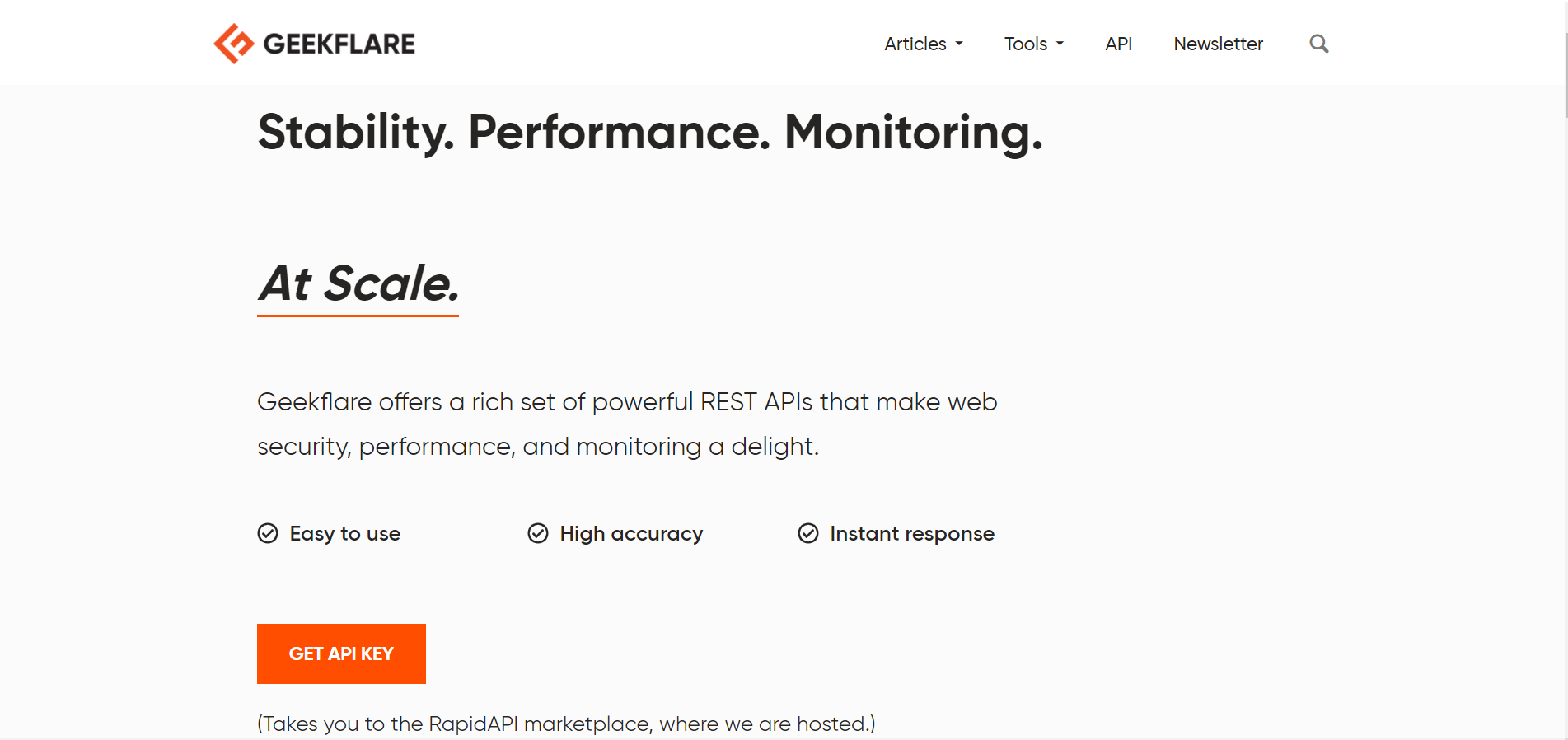
You can find different types of APIs including Is Site Up? API which we are interested in for this article. To use the Geekflare APIs we need an API Key that we can get through RapidAPI.
Click on the GET API KEY button to go to the RapidAPI.
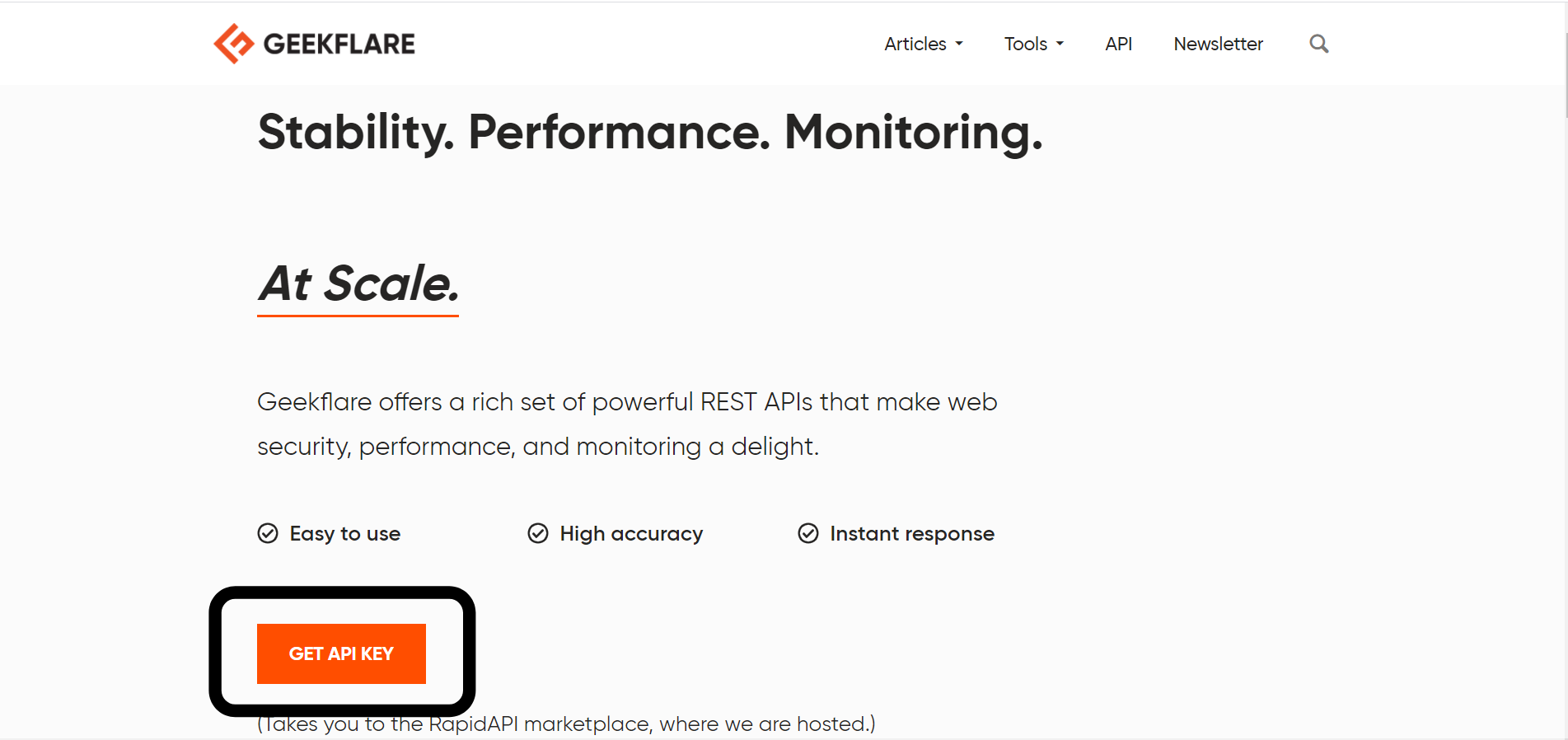
RapidAPI will open in a new tab as follows.

We need to create an account to get the API key. Create an account in RapidAPI if you don’t have one. Sign in to your account after creating an account.

You will find the Is Site Up? API at the top of all Geekflare APIs which we are looking for. If it’s not active find it using the search available and click on it.
You will get the usage of API on the right side. Select the Python with Requests from the Code Snippets section on the right side.
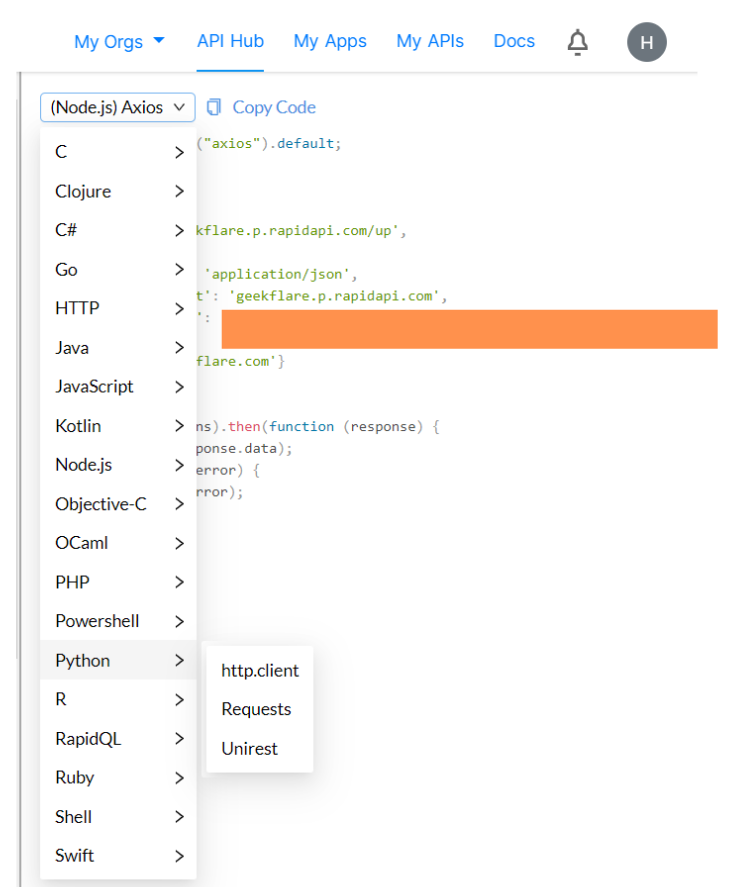
Choose your preferred language with the respective package if you are not using Python.
You will get the code to call the Is Site Up? API. Let’s modify it a bit which helps us to easily add more code later. Have look at the modified code in Python.
import requests
API_URL = "https://geekflare.p.rapidapi.com/up"
def make_api_request():
headers = {
'content-type': "application/json",
'x-rapidapi-host': "geekflare.p.rapidapi.com",
'x-rapidapi-key': "YOUR_API_KEY"
}
payload = r'{"url": "https://www.geekflare.com"}'
response = requests.request("POST", API_URL, data=payload, headers=headers)
return response.json()
if __name__ == '__main__':
data = make_api_request()
print(data)
Replace the API_KEY with your own API key from the RapidAPI in the above code. It will be different for every user. You will find it in the RapidAPI under the Headers Parameters section as follows.

You can find the same API key in the sample code as shown below.

Copy the key and add it to the above code
Multi-Location
The above code checks whether the site is up or not from a single location (New York, USA). But, we can test it from different locations with locations in the request body.
Other available locations are England (London) and Singapore. We can pass the locations data along with the site URL as follows.
{
"locations": [
"uk",
"us",
"sg"
],
"url": "geekflare.com"
}
You may pass the locations that you prefer from the list.
We have completed the code to make an API request that fetches the data whether a site is up or not. Now, it’s time to write some more code that sends a mail when the site is down. Let’s go.
Receiving email when the site is down
You can find the detailed tutorial on How to Send Emails through Gmail in Python Or use the following code which uses a package called yagmail specially designed to send mails from Gmail.
Before sending a mail through your Gmail account, we have to turn on the Allow less secure apps options. You can turn it on here.
Let’s see the code.
def send_mail():
gmail = yagmail.SMTP("gmail", "password")
receiver = "[email protected]"
subject = "Testing Subject"
body = "This is a testing mail"
gmail.send(
to=receiver,
subject=subject,
contents=body,
)
You can find the complete tutorial of yagmail here.
Now, we have code for API requests and sending mail. Our next step is to invoke the send_mail whenever we receive bad status from the API request.
So, how do we know that our site is down or up? When we request the Is Site Up? API, it will respond with some data as follows.
{'timestamp': 1629556759685,
'apiStatus': 'success',
'apiCode': 201,
'message': 'Site is up.',
'meta': {'url': 'https://www.geekflare.com',
'followRedirect': False,
'test': {'id': 'eu0frmah05mids55elkjgevkzd8ur3vk'}},
'data': [{'country': 'United States', 'city': 'New York',
'description': 'Site is up.',
'statusCode': 301, 'reasonPhrase': 'Moved Permanently'}]}
You will find a key called message in it. The value of the key message tells us whether the site is up or down. So, can be two types of messages as follows.
- Site is up.
- Site is down.
I think you got it now. So, we will send the mail when we receive the message “Site is down.”. The final code will look as follows.
import requests
import yagmail
API_URL = "https://geekflare.p.rapidapi.com/up"
def make_api_request():
headers = {
'content-type': "application/json",
'x-rapidapi-host': "geekflare.p.rapidapi.com",
'x-rapidapi-key': "API_KEY"
}
payload = r'{"url": "https://www.abcd.com"}'
response = requests.request("POST", API_URL, data=payload, headers=headers)
return response.json()
def send_mail(content):
gmail = yagmail.SMTP("gmail", "password")
receiver = "[email protected]"
subject = "Your Site is Down"
gmail.send(
to=receiver,
subject=subject,
contents=content,
)
if __name__ == '__main__':
data = make_api_request()
message = data['message']
## seding the mail
if message == 'Site is down.':
## extracting the errors from different locations
locations_data = data['data']
mail_content = "Your site is down due to unexpected error. See the useful data to resolve errors below.nn"
for location in locations_data:
mail_content = f"{location['city']}, {location['country']} - {location['error']}n"
mail_content = "nCheck the error and resolve them as soon as possible."
send_mail(mail_content)
You may update the content of the mail as you prefer. We have completed sending mail whenever our site is down. See the sample mail that has been received through the above code.
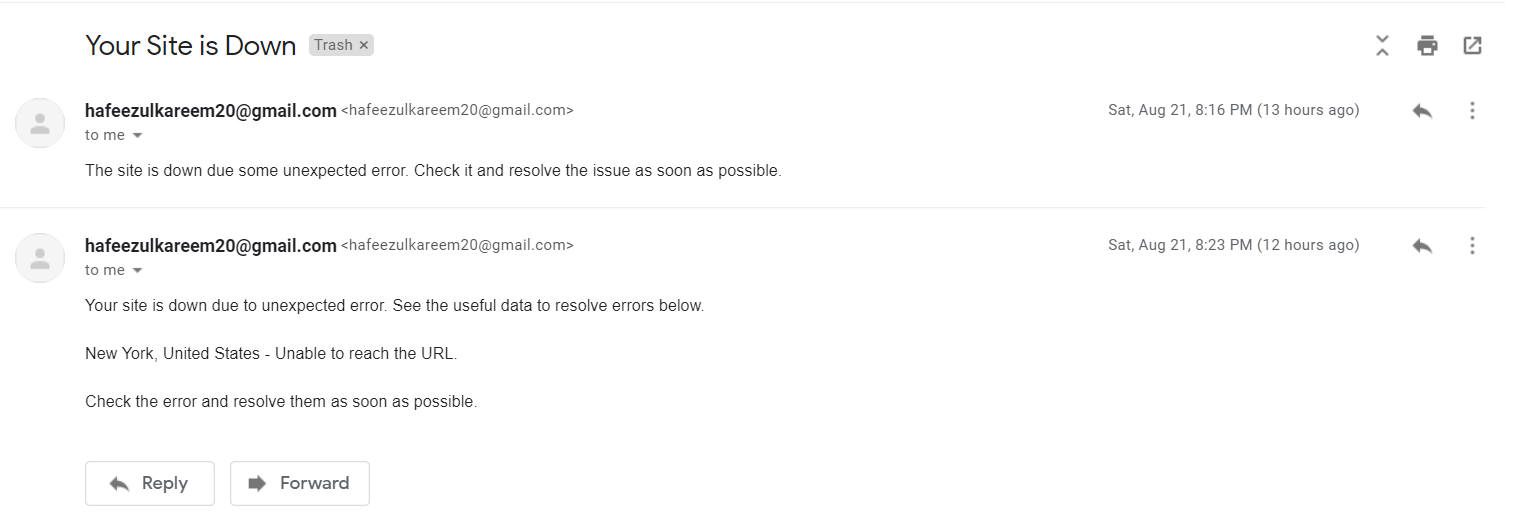
But, there is still a problem.
We have to execute our code to check whether our site is up or down. How often do we run it? Yeah, it depends on your preference. Let’s say we have to check it every one hour.
We can open a terminal or command line and execute our code every hour. But, it’s a repetitive process and boring one. And sometimes we can forget to check it. So, we need to execute the code automatically every hour.
Here we can make use of the cron to automatically execute our code every hour. Let’s see how to set up it.
Cron Setup
Let’s see the steps to set up the cron on a UNIX-based operating system.
- Open terminal.
- Run the command
crontab -e
which opens the crontab file in the terminal. - Press the key
i
to enter into the INSERT mode. - Now, add the cron pattern, Python directory and our code file directory. You see the example below.
0 * * * * /usr/bin/python3 /home/sample.py

We have set the pattern to execute the code every hour. But, you may need to set it to every minute based on the requirement. So, you can use the Crontab Guru or other cron tools to generate the cron pattern for the schedule.
That’s it. We have completed the set-up to get notified when the site is down.
Conclusion
Use the cron to schedule the script to run periodically on your cloud server that runs 24/7 to get notified through email when the site is down. Automation saves a lot of time and works for us. So, make use of it as we did in this article.
Happy Monitoring 🙂